What are the best ways to sort cryptocurrency arrays by value in PHP?

I am working on a project that involves sorting cryptocurrency arrays by value in PHP. Can anyone suggest the best ways to achieve this? I want to ensure that the sorting is accurate and efficient. Any insights or code examples would be greatly appreciated!
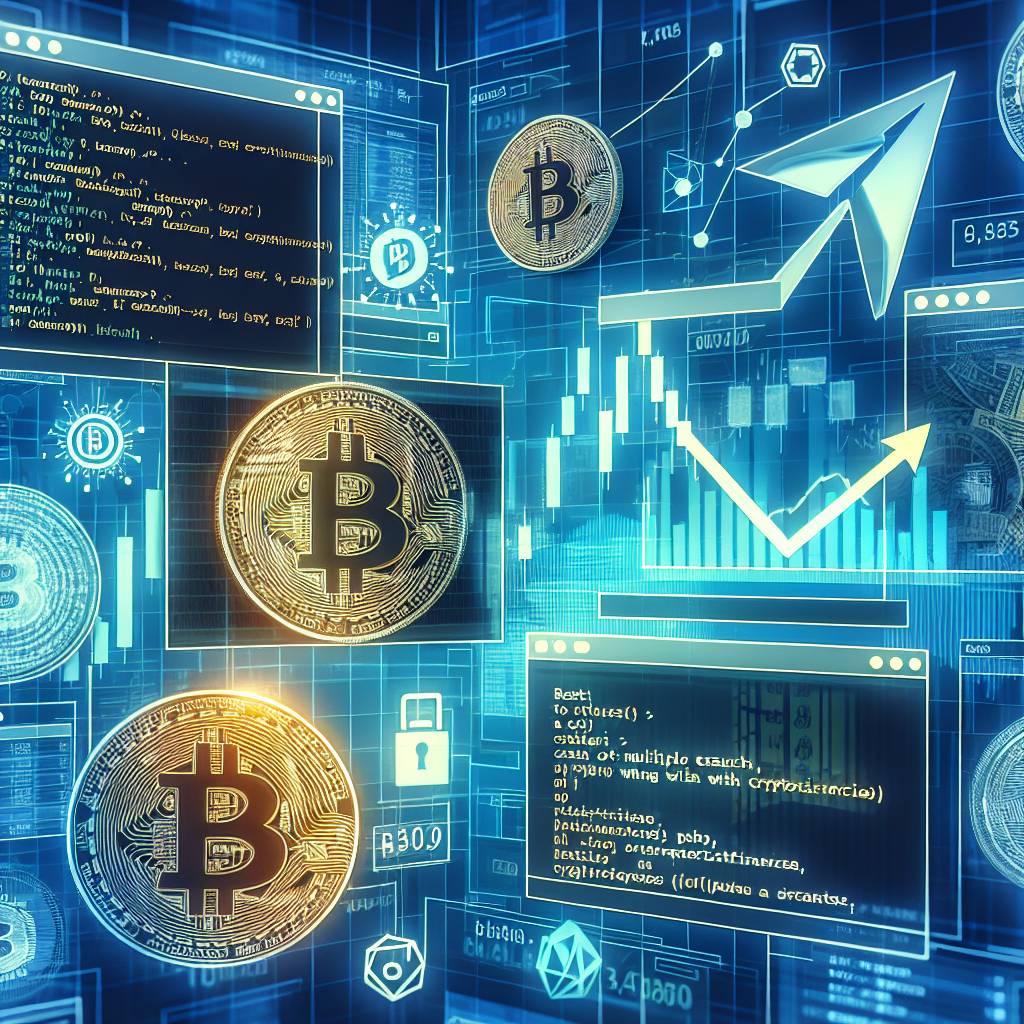
3 answers
- One of the best ways to sort cryptocurrency arrays by value in PHP is to use the built-in array_multisort() function. This function allows you to sort multiple arrays or a multi-dimensional array by one or more columns. You can specify the sorting order (ascending or descending) and the sorting type (numeric or string). Here's an example code snippet: $coins = array('Bitcoin', 'Ethereum', 'Ripple'); $values = array(10000, 2000, 500); array_multisort($values, SORT_DESC, $coins); This will sort the $coins array based on the corresponding values in the $values array, in descending order. Another option is to use the usort() function, which allows you to define a custom comparison function. You can use this function to compare the values of the cryptocurrencies and sort the array accordingly. Here's an example code snippet: $coins = array( array('name' => 'Bitcoin', 'value' => 10000), array('name' => 'Ethereum', 'value' => 2000), array('name' => 'Ripple', 'value' => 500) ); usort($coins, function($a, $b) { return $b['value'] - $a['value']; }); This will sort the $coins array based on the 'value' key in descending order. Hope this helps!
Dec 25, 2021 · 3 years ago
- Sorting cryptocurrency arrays by value in PHP can be done using the array_multisort() function. This function allows you to sort multiple arrays or a multi-dimensional array by one or more columns. You can specify the sorting order (ascending or descending) and the sorting type (numeric or string). Another option is to use the usort() function, which allows you to define a custom comparison function. You can use this function to compare the values of the cryptocurrencies and sort the array accordingly. Both methods are efficient and widely used in PHP programming. Happy coding!
Dec 25, 2021 · 3 years ago
- When it comes to sorting cryptocurrency arrays by value in PHP, one of the best ways is to use the array_multisort() function. This function allows you to sort multiple arrays or a multi-dimensional array by one or more columns. You can specify the sorting order (ascending or descending) and the sorting type (numeric or string). Another option is to use the usort() function, which allows you to define a custom comparison function. You can use this function to compare the values of the cryptocurrencies and sort the array accordingly. Both methods are commonly used in PHP development and provide efficient sorting capabilities. Give them a try and see which one works best for your project!
Dec 25, 2021 · 3 years ago
Related Tags
Hot Questions
- 83
How can I buy Bitcoin with a credit card?
- 81
What is the future of blockchain technology?
- 76
How can I minimize my tax liability when dealing with cryptocurrencies?
- 61
What are the best digital currencies to invest in right now?
- 56
How does cryptocurrency affect my tax return?
- 56
What are the advantages of using cryptocurrency for online transactions?
- 44
How can I protect my digital assets from hackers?
- 35
What are the tax implications of using cryptocurrency?